首先看效果:
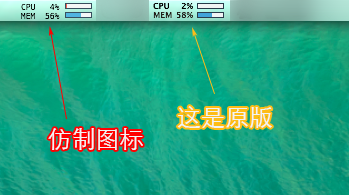
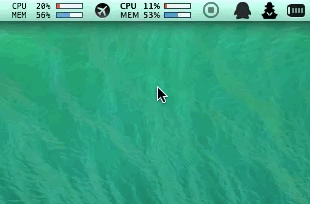
下面是所用到的颜色:
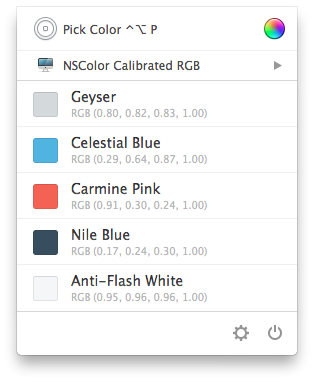
分析:
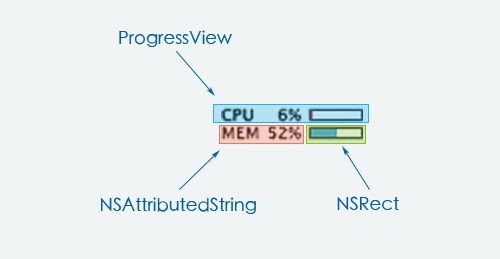
代码
因为是demo,所以很多地方的代码组织不完善。
//
// StatusItemView.h
// SysStatus
//
// Created by xiaozi on 14-2-23.
// Copyright (c) 2014年 xiaozi. All rights reserved.
//
#import <Cocoa/Cocoa.h>
#import "ProgressView.h"
@interface StatusItemView : NSControl <NSMenuDelegate> {
NSStatusItem *_statusItem;
BOOL _isHighlighted;
NSDictionary *_data;
SEL _action;
id __unsafe_unretained _target;
ProgressView *_cpuView;
ProgressView *_memView;
}
@property (nonatomic, readonly) NSStatusItem *statusItem;
@property (nonatomic, setter = setHighlighted:) BOOL isHighlighted;
@property (nonatomic) NSDictionary *data;
@property (nonatomic) SEL action;
@property (nonatomic, unsafe_unretained) id target;
- (id) initWithStatusItem: (NSStatusItem *)statusItem;
@end
//
// StatusItemView.m
// SysStatus
//
// Created by xiaozi on 14-2-23.
// Copyright (c) 2014年 xiaozi. All rights reserved.
//
#import "StatusItemView.h"
#import "ProgressView.h"
@implementation StatusItemView
- (id) initWithStatusItem: (NSStatusItem *)statusItem
{
self = [super init];
if (self) {
// Initialization code here.
_statusItem = statusItem;
_cpuView = [[ProgressView alloc] initWithFrame: NSMakeRect(0, 10, 82, 11)];
[_cpuView setBarColor:[NSColor colorWithCalibratedRed:0.91 green:0.3 blue:0.24 alpha:1]];
[_cpuView setData:[NSDictionary dictionaryWithObjectsAndKeys:@"CPU %3d%%", @"label", [NSNumber numberWithInt: 0], @"value", nil]];
_memView = [[ProgressView alloc] initWithFrame: NSMakeRect(0, 1, 82, 11)];
[_memView setBarColor:[NSColor colorWithCalibratedRed:0.29 green:0.64 blue:0.87 alpha:1]];
[_memView setData:[NSDictionary dictionaryWithObjectsAndKeys:@"MEM %3d%%", @"label", [NSNumber numberWithInt: 0], @"value", nil]];
[self addSubview:_cpuView];
[self addSubview:_memView];
}
return self;
}
- (void) setData: (NSDictionary *)data
{
[_cpuView setData:[NSDictionary dictionaryWithObjectsAndKeys:@"CPU %3d%%", @"label", [data objectForKey: @"cpu"], @"value", nil]];
[_memView setData:[NSDictionary dictionaryWithObjectsAndKeys:@"MEM %3d%%", @"label", [data objectForKey: @"mem"], @"value", nil]];
}
- (void) setHighlighted: (BOOL) highlighted
{
if (_isHighlighted == highlighted) return;
_isHighlighted = highlighted;
[_cpuView setHighlighted: highlighted];
[_memView setHighlighted: highlighted];
[self setNeedsDisplay: YES];
}
- (void) setMenu:(NSMenu *)menu
{
[menu setDelegate: self];
[super setMenu: menu];
}
- (void)mouseDown:(NSEvent *)theEvent
{
[_statusItem popUpStatusItemMenu: [super menu]];
[NSApp sendAction:_action to:_target from:self];
}
- (void)menuWillOpen:(NSMenu *)menu {
[self setHighlighted:YES];
[self setNeedsDisplay:YES];
}
- (void)menuDidClose:(NSMenu *)menu {
[self setHighlighted:NO];
[self setNeedsDisplay:YES];
}
- (void)drawRect:(NSRect)dirtyRect
{
[_statusItem drawStatusBarBackgroundInRect:dirtyRect withHighlight: _isHighlighted];
}
@end
//
// ProgressView.m
// SysStatus
//
// Created by xiaozi on 14-2-24.
// Copyright (c) 2014年 xiaozi. All rights reserved.
//
#import "ProgressView.h"
@implementation ProgressView
- (id)initWithFrame:(NSRect)frame
{
self = [super initWithFrame:frame];
if (self) {
// Initialization code here.
_data = [NSDictionary dictionaryWithObjectsAndKeys:@"LAB %3d%%", @"label", [NSNumber numberWithInt: 50], @"value", nil];
_barColor = [NSColor colorWithCalibratedRed:0.91 green:0.3 blue:0.24 alpha:1];
}
return self;
}
- (void) setHighlighted:(BOOL)highlighted
{
if (_highlighted == highlighted) return;
_highlighted = highlighted;
[self setNeedsDisplay: YES];
}
-(void) setData:(NSDictionary *)data
{
_data = data;
[self setNeedsDisplay: YES];
}
- (void)drawRect:(NSRect)dirtyRect
{
NSColor *fontColor,*barBorderColor,*barIndicatorColor,*barBgColor;
if (_highlighted) {
fontColor = [NSColor whiteColor];
barBorderColor = [NSColor whiteColor];
barIndicatorColor = [NSColor whiteColor];
barBgColor = [NSColor clearColor];
} else {
fontColor = [NSColor blackColor];
barBorderColor = [NSColor colorWithCalibratedRed:0.17 green:0.24 blue:0.3 alpha:1];
barIndicatorColor = _barColor;
barBgColor = [NSColor colorWithCalibratedRed:0.95 green:0.96 blue:0.96 alpha:1];
}
NSDictionary *attributes = [NSDictionary dictionaryWithObjectsAndKeys:[NSFont fontWithName:@"Menlo" size: 8], NSFontAttributeName, fontColor, NSForegroundColorAttributeName, nil];
NSAttributedString * text=[[NSAttributedString alloc] initWithString:[NSString stringWithFormat: [_data valueForKey:@"label"], [[_data objectForKey: @"value"] integerValue]] attributes: attributes];
[text drawAtPoint:NSMakePoint(6, 1)];
NSRect bar = NSMakeRect(50.0f, 3, 27.0f, 6.0f);
bar = NSInsetRect(bar, .5f, .5f);
NSBezierPath *barView = [NSBezierPath bezierPathWithRect: bar];
[barBgColor set];
[barView fill];
[barBorderColor set];
[barView stroke];
NSRect barIndicator = NSMakeRect(50.0f, 3, roundf(.27f * [[_data objectForKey: @"value"] integerValue]), 6.0f);
barIndicator = NSInsetRect(barIndicator, 1, 1);
[barIndicatorColor set];
[NSBezierPath fillRect:barIndicator];
}
@end
使用方法
- (void)applicationDidFinishLaunching:(NSNotification *)aNotification
{
// Insert code here to initialize your application
NSStatusItem *statusItem = [[NSStatusBar systemStatusBar] statusItemWithLength:82];
statusItemView = [[StatusItemView alloc] initWithStatusItem: statusItem];
[statusItem setHighlightMode: YES];
[statusItem setView: statusItemView];
[statusItemView setMenu: _statusMenu];
[NSTimer scheduledTimerWithTimeInterval:2
target:self
selector:@selector(updateInfo:)
userInfo:nil
repeats:YES];
}
- (void)updateInfo:(NSTimer *)timer
{
int cpuUsage = (arc4random() % 20) + 2;
int memUsage = (arc4random() % 10) + 50;
// NSLog(@"%d, %d", cpuUsage, memUsage);
NSDictionary *data = [NSDictionary dictionaryWithObjectsAndKeys:[NSNumber numberWithInt: cpuUsage], @"cpu", [NSNumber numberWithInt: memUsage], @"mem", nil];
[statusItemView setData: data];
}