前提
需要一个 http服务器提供访问,还需要一个 websocket吐服务器数据,至于怎么编译nginx这边就不讲了
预览
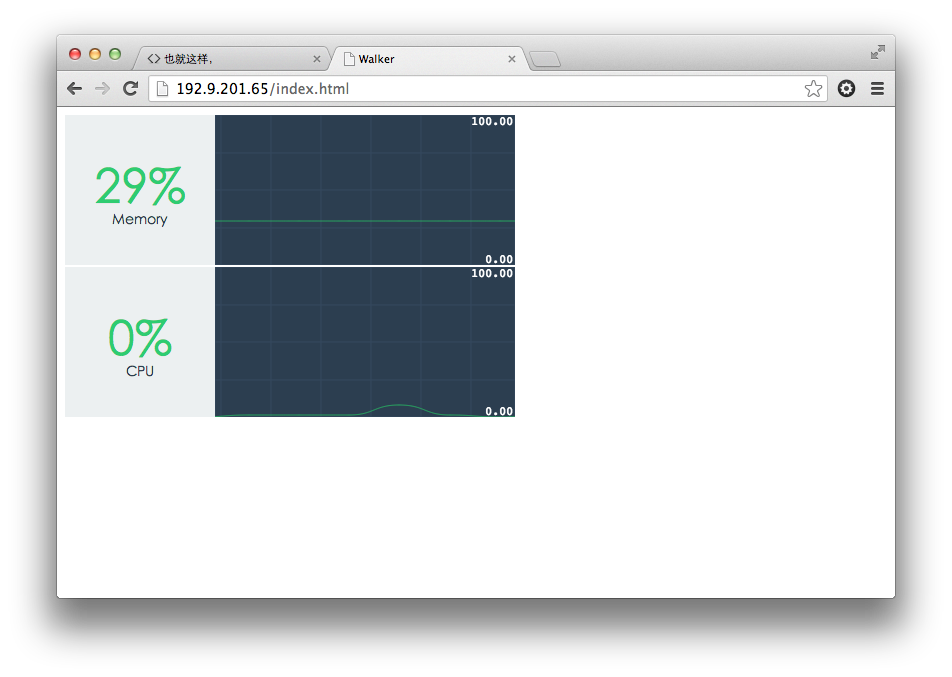
代码
这里主要给出后端python的代码,前端主要是用backbone + smoothiecharts
实现
#!/usr/bin/env python
# encoding: utf-8
import tornado.web
import tornado.websocket
import tornado.ioloop
import subprocess, threading, json, re
def vmstat():
memoryKey = ['swpd', 'free', 'buff', 'cache', 'total']
cpuKey = ['us', 'sy', 'id', 'wa']
space = re.compile('\s+')
memTotal = subprocess.check_output("cat /proc/meminfo | grep MemTotal | sed 's/\\w*:\\s*\\([0-9]*\\).*/\\1/'", shell = True).strip()
# print(memTotal)
# 这个地方要使用 shell = True 的话,需要记得退出的时候关闭子进程
p = subprocess.Popen(['vmstat', '1', '-n'], stdout = subprocess.PIPE)
io_loop = tornado.ioloop.IOLoop.instance()
p.stdout.readline()
p.stdout.readline()
for line in iter(p.stdout.readline, ''):
# print(line)
fields = space.split(line.strip())
memoryVal = fields[2: 6]
memoryVal.append(memTotal)
result = dict(memory=dict(zip(memoryKey, memoryVal)), cpu=dict(zip(cpuKey, fields[12: 16])))
result = json.dumps(result)
print(result)
for waiter in MonitorHandler.waiters:
io_loop.add_callback(waiter.write_message, result)
class MonitorHandler(tornado.websocket.WebSocketHandler):
waiters = set()
def open(self):
MonitorHandler.waiters.add(self)
print('waiters: ', len(MonitorHandler.waiters))
def on_message(self, message):
pass
def on_close(self):
MonitorHandler.waiters.remove(self)
print('waiters: ', len(MonitorHandler.waiters))
class Application(tornado.web.Application):
def __init__(self):
handlers = [
('/', MonitorHandler)
]
super(Application, self).__init__(handlers)
def main():
app = Application()
app.listen(8888)
t = threading.Thread(target=vmstat)
t.daemon = True
t.start()
print('Starting the server...')
tornado.ioloop.IOLoop.instance().start()
if __name__ == '__main__':
main()